Mouse Hover Image Zoom Effect Animation Using CSS
Creating a zoom effect animation an image when the mouse hovers over it can be achieved using CSS. This effect is often used by e-commerce websites to show more detail in an image. Using CSS properties such as transform and transition, the image can smoothly zoom in or out when the mouse hovers over it.
Hover Image Zoom Effect Animation
Let's explore how to create a zoom effect animation when hovering over an image. This tutorial will provide the complete HTML and CSS code, along with a working demonstration. By following these steps.
Demo
Hover your mouse over the images to see the zooming animation.
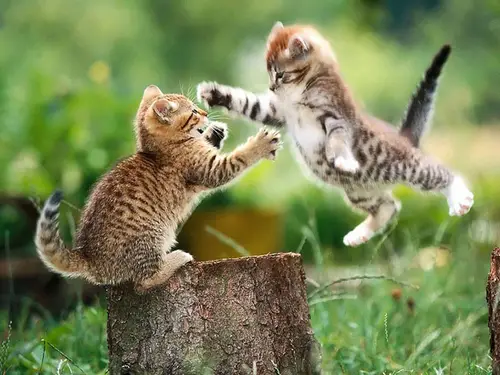
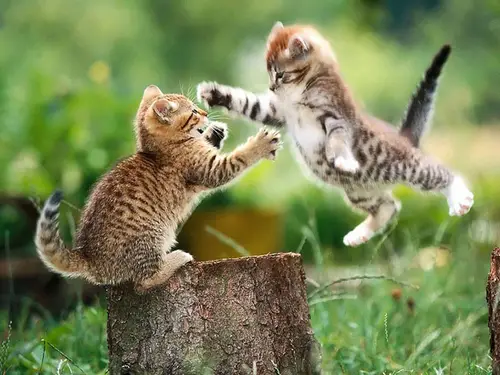
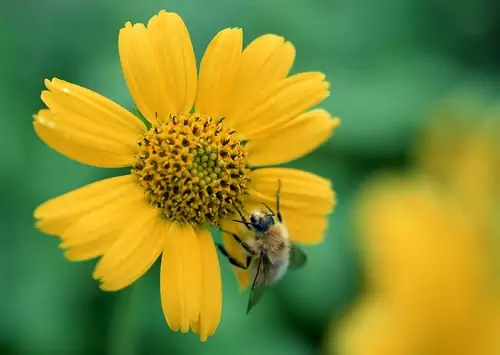
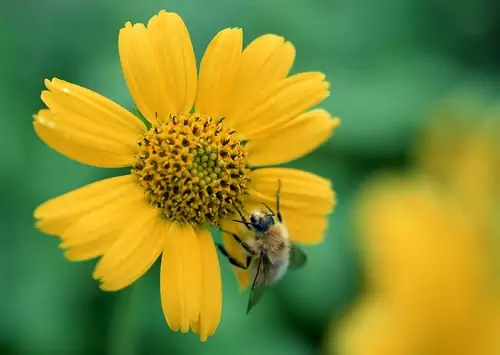
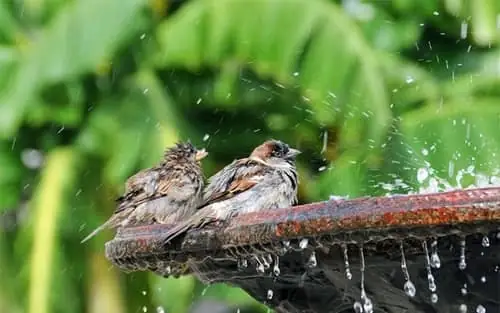
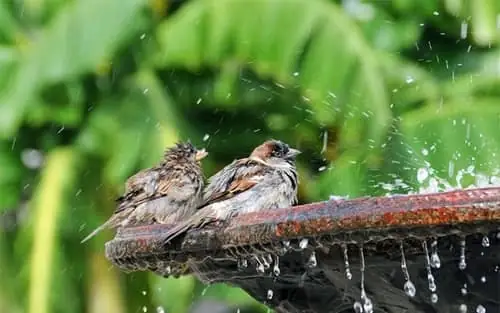
How to Create This Animation
Create a <div>
tag and inside it two <img>
tags. Set a small image for the first image tag. For the second image, put a larger size image. Apply the provided CSS animation code to animate the second image as shown below. When you hover your mouse over the first image, you will see the zoom-in effect of the second image.
<div class="zoom-effect">
<img class="img-small" src="/images/demo/3.webp" alt="image">
<img class="img-zoom-animation" src="/images/demo/3.webp" alt="image">
</div>
.img-small {
width: 100px;
}
.img-zoom-animation {
position: absolute;
width: 0px;
z-index: 10;
transition: width 0.3s linear 0s;
}
.img-small:hover+.img-zoom-animation {
width: 400px; /* maximum zoom width */
}
Design a Real-Time CSS Image Zoom Animation
Insert your image and apply a zoom animation effect.
Instructions for Using This Tool
- Upload your image or provide an image URL.
- Adjust the image size to your preference.
- Adjust the zoom level and animation duration.
- Copy and paste the generated code into your website.
Generated code HTML and CSS
<div class="zoom-effect">
<img class="img-small" src="/images/demo/3.webp" alt="image">
<img class="img-zoom-animation" src="/images/demo/3.webp" alt="image">
</div>
.img-small {
width: 100px;
}
.img-zoom-animation {
position: absolute;
width: 0px;
z-index: 10;
transition: width 0.3s linear 0s;
}
.img-small:hover+.img-zoom-animation {
width: 400px;
}
To make changes image style, click the button below to edit and preview them live
How To Use This Animation For Multiple Images
When using more images for this zoom animation, sometimes large image takes longer to load that affect your page loading performance. So you need to modify your HTML codes and here is the solution for that.
Modify the HTML code to include an attribute 'data-big
' within the second <img>
tag and assign the URL of the big image URL to the 'data-big
' attribute.
<div class="zoom-effect">
<img class="img-small" src="/images/small-image1.png" alt="image">
<img class="img-zoom-animation" src="/images/small-image1.png" data-big="/images/big-image1.png" alt="image">
</div>
<div class="zoom-effect">
<img class="img-small" src="/images/small-image2.png" alt="image">
<img class="img-zoom-animation" src="/images/small-image2.png" data-big="/images/big-image2.png" alt="image">
</div>
<div class="zoom-effect">
<img class="img-small" src="/images/small-image3.png" alt="image">
<img class="img-zoom-animation" src="/images/small-image3.png" data-big="/images/big-image3.png" alt="image">
</div>
Using JavaScript, replace the 'src
' value of the second image tag and add the value of the 'data-big
' attribute.